Java Tutorials
Next up in our series exploring functional programming in java 8, the map method.
Read nowReduce is a functional programming technique that combines values and returns a value. Find out how to use the stream reduce function in java 8.
Read nowLets look at an example of a predicate that filters even numbers then we will use the same predicate to filter odd numbers
Read nowFind out how java 8 provided native support for grouping object by attribute
Read now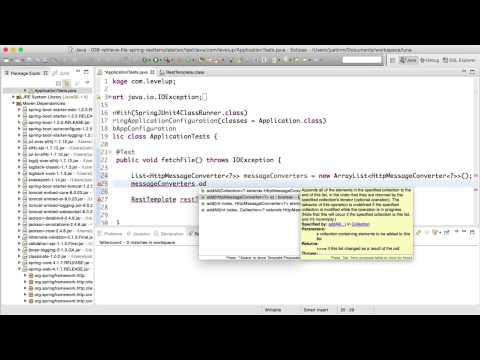
Whether it is image, pdf or word document find out how to download a file with Spring's RestTemplate
Watch Now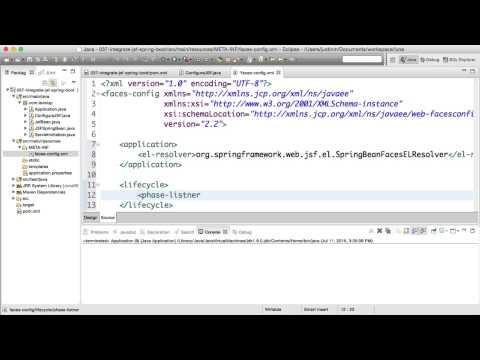
Learn how to integrate JSF with spring boot to allow common configuration across applications
Watch Now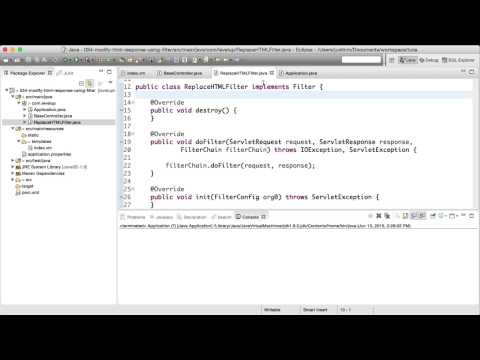
There may be instances where you want to modify the response before it is written to the client. In this tutorial we will show how to write a regex to append to a html element.
Watch Now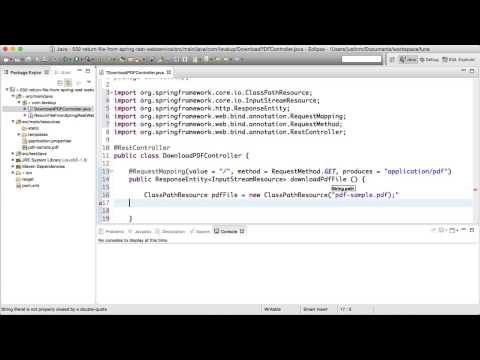
It is easy serving a static asset from a spring web application but what if you needed to fetch a file from a database or a file system? In this episode find out how to return a file from a spring controller.
Watch Now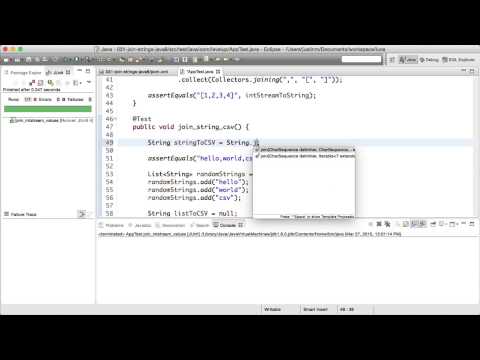
Just about every programming language natively supports a string join() function. Find out in this tutorial how to java 8 finally added support to join strings.
Watch Now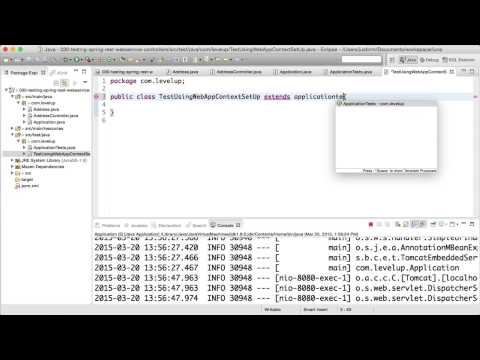
If you are not all in with test driven development and you built your spring
Watch Now@Controllers
before writing a test, find out in this screencast two methods for testing your rest endpoint by using spring mvc test framework.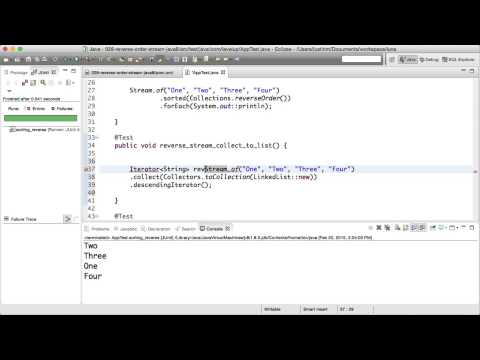
In this episode we will show how to reverse the element position while maintaining order in a java 8 stream.
Watch Now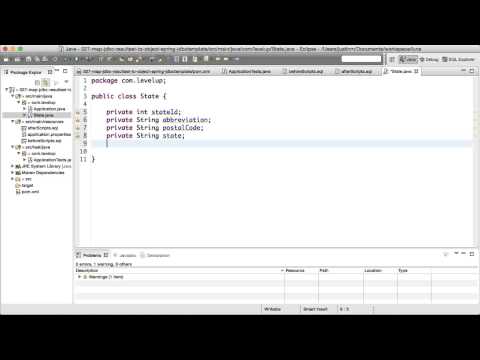
Object relational mappers allow you to map a lower level sql query to a java object. While spring's JdbcTemplate isn't considered a ORM, lets find out how to map a sql result set to a domain object.
Watch Now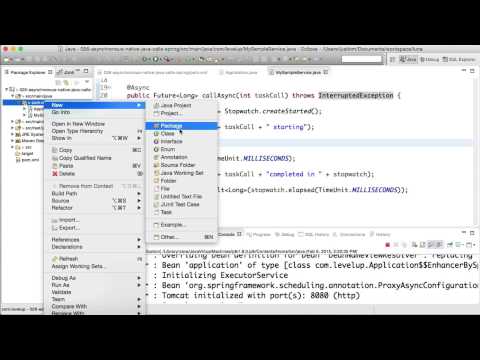
As consumers of websites demand fast page rendering times we as engineers need to continue to look for ways to make systems perform. Let us examine how to make asynchronous native java calls within a spring container.
Watch Now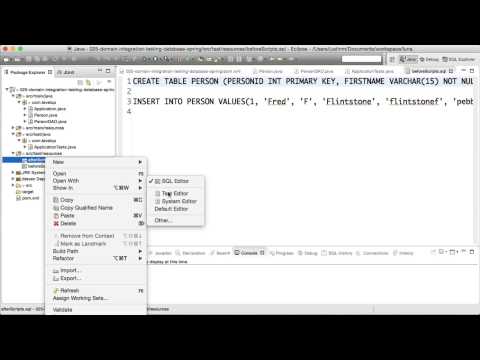
In episode 22 we how to seed a database with spring's @Sql annotation so lets extend it and find out how to test a domain with database interactions.
Watch Now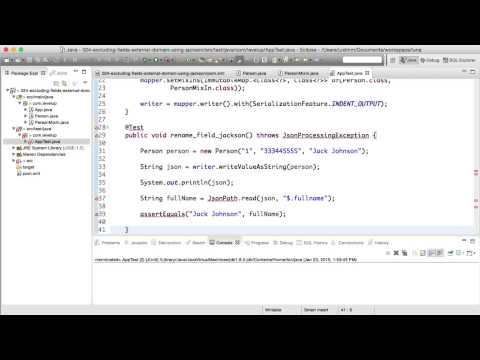
When you consume a third party library or an internal object from another business unit you may not have the ability to modify source code to add @JsonIgnore annotation. Let's find out how to use jacksons mixins to exclude and rename properties during the serialization and deserialization process.
Watch Now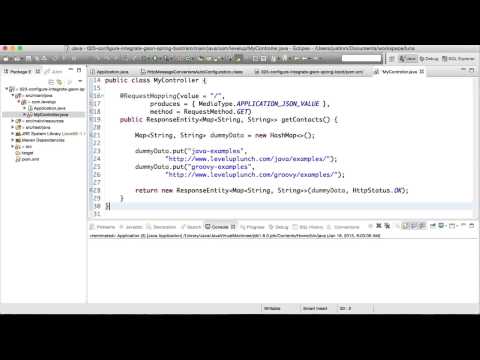
Jackson has been the default json library in springframework until version 4.1 where it added support to use Gson by configuring GsonHttpMessageConverter. Let's take a look at how to configure your spring application to use Google Gson library's Gson class.
Watch Now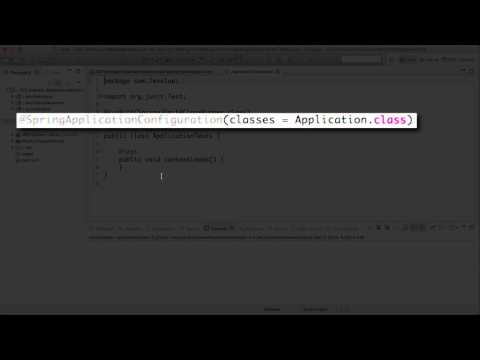
As agile penetrates into the enterprise more focus will be put on automated integration testing. Since many interactions with a domain require data in a constant state lets explore how to preload a local data store while running in spring context.
Watch Now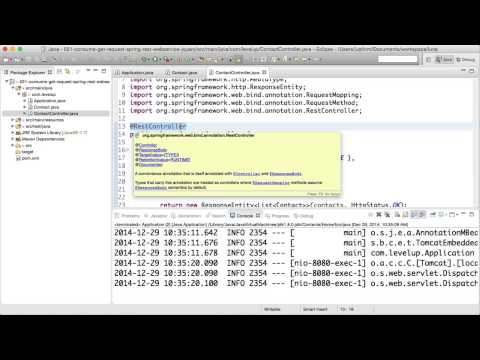
Exposing JSON from a spring controller is surprisingly simple. In this episode I will create a controller that returns JSON and make a GET request from a client side template using jquery.
Watch Now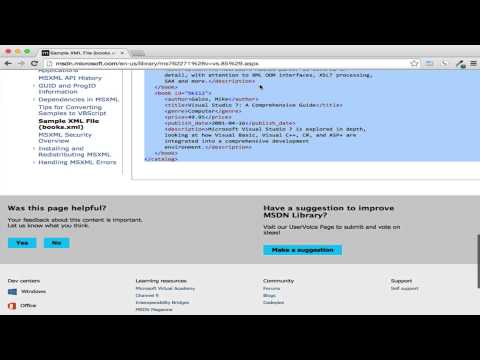
XSLT provides a way to transform a XML document into various format types. In this screencast find out how to convert XML into HTML using a java based XSLT transformer.
Watch Now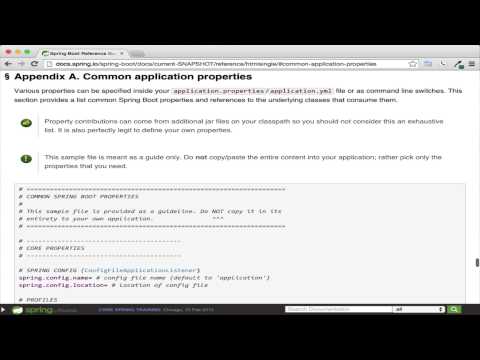
Enabling actuator provides many benefits but could expose internal system information even to an authenticated user. In this screencast we will show how to disable actuator endpoints from your spring boot application.
Watch Now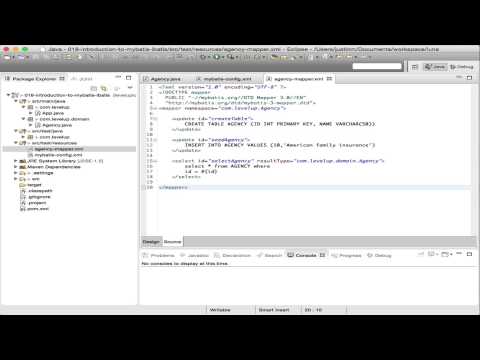
Using straight up jdbc data access is tedious, error prone and difficult to map database structures to object oriented types. In this tutorial we will explore how to get started with a persistence framework named mybatis.
Watch Now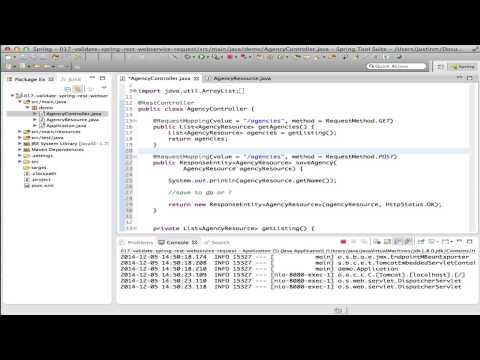
Find out how to validate a RESTFul request when posting to a spring controller using the bean validation API and spring's validator interface.
Watch Now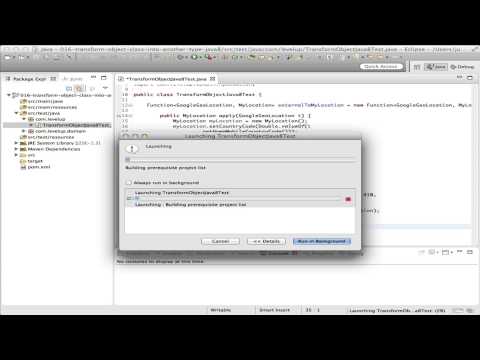
Quite often when calling to get external data you need to convert an object returned into a internal class. Learn how to transform an object into another type by using java 8 function and stream's map.
Watch Now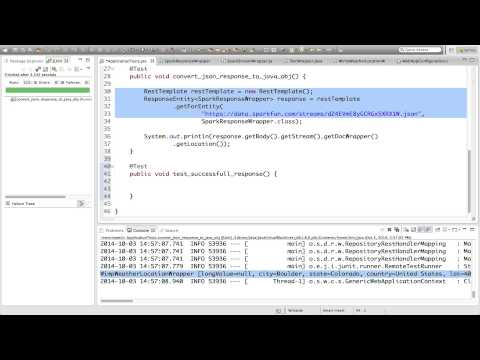
In this screencast we will walk through how to call a RESTFul webservice in java with spring's RESTTemplate.
Watch Now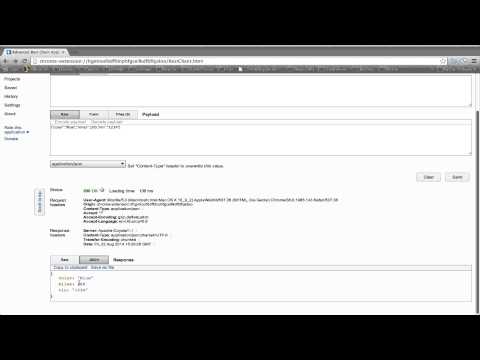
After making a GET request to a REST service the natural progression is to POST information back to the server. In this episode we will look at how to post json to spring controller and have it automatically convert JSON to arraylist, object or multiple objects.
Watch Now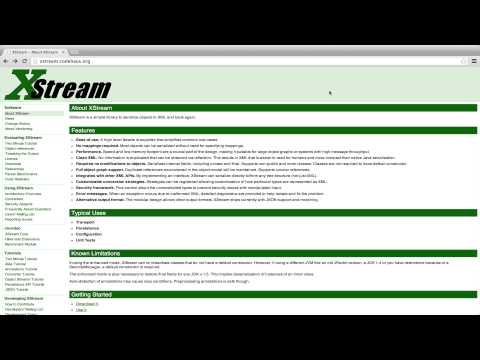
Returning JSON from a REST services is set up by default within Spring Boot. We will look at how to set up Spring Boot to return XML as the response.
Watch Now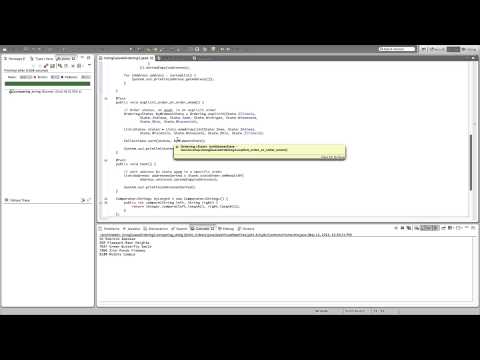
In Ordering part 1 we showed the basics. In this screencast we will show how to sort an arraylist of objects by a field, order an enum in explicit order, chain multiple operations and combine comparators.
Watch Now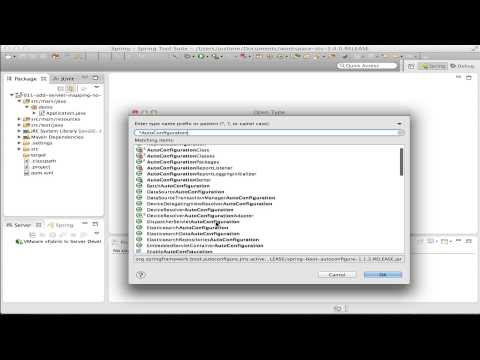
DispatchServlet is front controller that routes traffic to controllers and various components within Spring. Here I will show how to change the dispatch servlet-mapping in spring boot and servlet 3.0.
Watch Now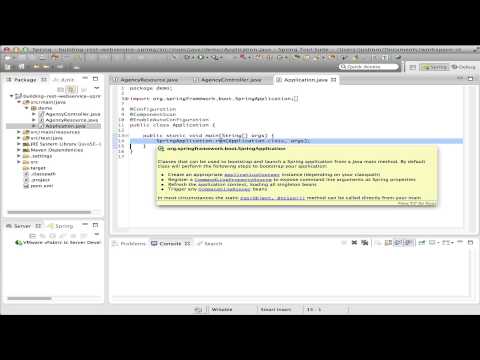
A RESTful service is a way to expose your data through a URL. Let's use spring mvc to implement a RESTFul webservice to retrieve a listing of agencies.
Watch Now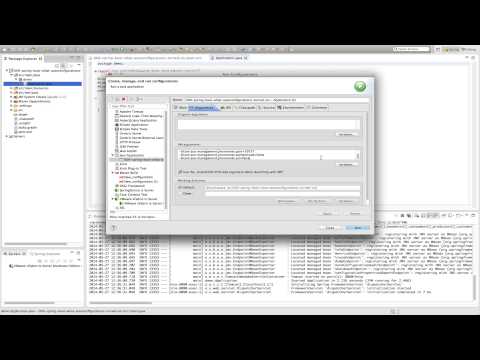
Since spring boot's convention over configuration makes assumptions with AutoConfiguration, it sometimes can be difficult troubleshooting what is turned on or turned off. This episode will show how to display a listing of configurations enabled within your application.
Watch Now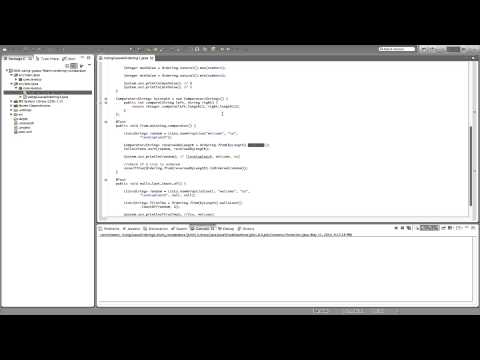
Guava ordering is a powerhouse class that simplifies using java comparator interface. In part one we will show basics on how call reverse, find the min and max, create Ordering object from existing comparator and position nulls within a list.
Watch Now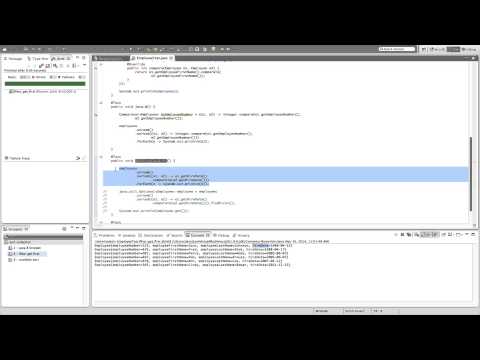
In Java 8 sorting has been simplified by removing the verbose comparator code and anonymous inner classes. Lets take a look at how making it easier could drive a different behavior among java developers.
Watch Now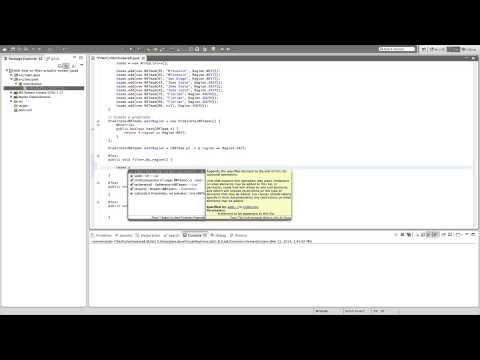
Almost every java application uses a collection and performs a filtering operation. With java 8 you can throw away those hard to read non portable for loops and take advantage of the Streams API.
Watch Now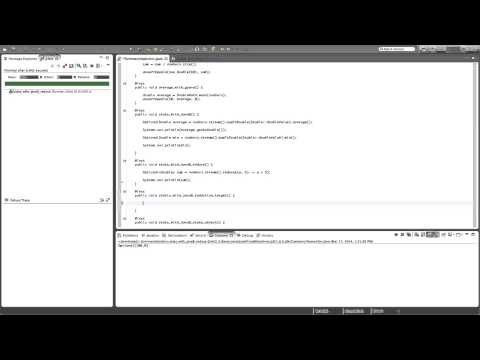
Java 8 has simplified finding summary statistics such as average, maximum, minimum, sum and count on collections and arrays. Using the Stream API the reduction operation is performed internally providing a clean and eloquent way to its predecessor for loop.
Watch Now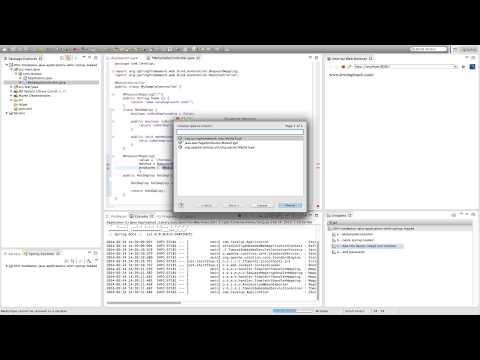
Spring-loaded enables hot deployments for your applications which allows you to increase productivity by reducing developer cycle time.
Watch Now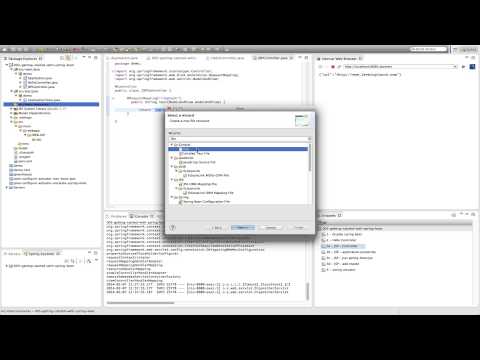
Tired of battling environments, xml hell and dealing with the same configuration files through out applications? Spring provides an opinionated way to facilitate application development with spring-boot.
Watch Now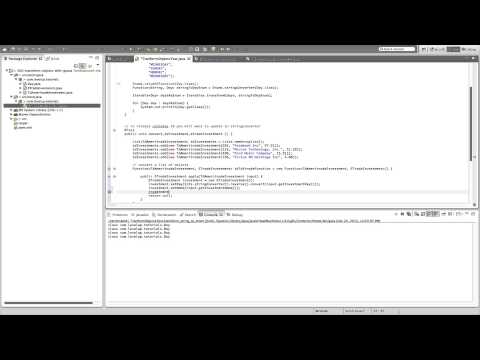
Working with multiple systems require mapping of data from one object to another of different types. Let us take a look at a lightweight way to convert objects utilizing guava functions
Watch Now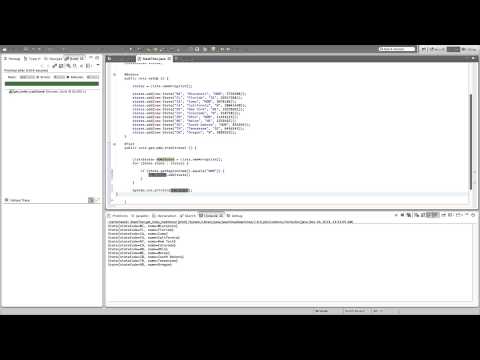
Filtering objects from a collection in java can be difficult to test and hard to read. Let's examine a cleaner approach to those multi-line if statements with Collections2.filter, a Google guava utility class.
Watch Now